This is an ongoing project... nothing is ever finished when you are learning 🌱
I wanted to revisit the nitty gritty basics of Python and share progress of a personal project. This is to emphasize that learning takes more than just completing an online course, you will maximize your abilities if you apply the knowledge and topics to a personal project.
I love food and cooking, so I wanted to see if I could create some kind of program that will cook different kinds of meats.
First, I created a dictionary with the meats I want to cook.
cookbook = {
"poultry" : {
"animal" : "chicken",
"cooked_temperature" : 165
},
"steak" : {
"animal" : "cow",
"cooked_temperature" : 145
},
"pork" : {
"animal" : "pig",
"cooked_temperature" : 145
},
"ground_beef" : {
"animal" : "cow",
"cooked_temperature" : 160
},
"fish" : {
"animal" : "fish",
"cooked_temperature" : 145
}
}
Off to the kitchen! 👩🏻🍳
I started writing my program with a focus on Chicken 🐔🍗
This starts very simple, defining variables and creating an if/else statement.
x = 165
y = 'chicken'
if y == 'chicken' and x < 165 :
print("The chicken is not done")
else :
print("The chicken is ready to eat!")
It is very important that you define your variables with descriptive names. DO NOT use 'x' and 'y' like I did above, that does not help. You want to write code in a way that other people can read it and know what it does (so long as they know how to read Python) 🤓
degrees_fahrenheit = 165
meat_type = 'chicken'
if meat_type == 'chicken' and degrees_fahrenheit < 165 :
print("The chicken is not done")
else :
print("The chicken is ready to eat!")
This brings in a while loop, which increments the temperature through each iteration.
The chicken starts at a temperature of 75 degrees, which assumes that you start cooking your meat at room temperature.
⚠ This probably is not a good idea for chicken but if preferable for beef.
If the temperature is still below the cooked temperature, then it prints 'Put me back in! I am still raw'
One the chicken has reached safety regulated temperature of at least 165 degrees, it prints 'Stick a fork in me, I am done!'
🧠 Improvement Opportunities:
- Specify the amount of time each iteration takes and be more accurate with how to increment the temperature (below uses 12 for no real reason)
current_temp = 75 # assumes you start cooking your meat at room temperature
chicken_cooked = 165 # safe temperature for cooked chicken
meat_type = 'chicken'
if meat_type == 'chicken' :
while True :
if current_temp < chicken_cooked :
print('The chicken is currently ' + str(current_temp) + ' degrees fahrenheit')
print('Put me back in! I am still raw')
elif current_temp == chicken_cooked :
print('The chicken is currently ' + str(current_temp) + ' degrees fahrenheit')
print('Stick a fork in me, I am done!')
elif current_temp > chicken_cooked :
print('Oh no! I am overcooked by ' + str(current_temp - chicken_cooked) + ' degrees')
break
current_temp = current_temp + 12
For fun, this was my first function attempted outside of my formal course.
As seen in the notes, the purpose of this function is to create a sentence when passed two strings as parameters.
def my_function(word1,word2): # this is a comment labeling the function header
''' The purpose of my function is to create a sentence when passed
two strings as parameters '''
sentence = word1 + ' ' + word2 + '!'
return(sentence)
abracadabra = my_function('Hello','World')
print(abracadabra)
I am slowly starting to introduce more types of meat. I did this by creating a function. You can see by the notes that this function takes a string parameter and checks the cooking time for the type of meat.
The same while loop is used here but with a new concept of global vs. local variables.
👉🏻 chicken_cooked and pork_cooked are global variables (they can be used throughout the entire script.
👉🏻 current_temp is a local variable (it can only be used within the function)
🧠 Improvement Opportunity:
- I am sure there is a way to create parameters within the function instead of having two of the same loops for each meat. This is not sustainable / scalable.
chicken_cooked = 165 # safe temperature for cooked chicken
pork_cooked = 145 # safe temperature for cooked pork
def cooking(meat_type):
''' This function takes a sting parameter and checks the cooking time for the type of meat '''
current_temp = 75 # assumes you start cooking your meat at room temperature
if meat_type == 'chicken':
while True :
if current_temp < chicken_cooked :
print('The chicken is currently ' + str(current_temp) + ' degrees fahrenheit')
print('Put me back in! I am still raw')
elif current_temp == chicken_cooked :
print('The chicken is currently ' + str(current_temp) + ' degrees fahrenheit')
print('Stick a fork in me, I am done!')
elif current_temp > chicken_cooked :
print('Oh no! I am overcooked by ' + str(current_temp - chicken_cooked) + ' degrees')
break
current_temp = current_temp + 12
elif meat_type == 'pork':
while True :
if current_temp < pork_cooked :
print('The pork is currently ' + str(current_temp) + ' degrees fahrenheit')
print('Put me back in! I am still raw')
elif current_temp == pork_cooked :
print('The pork is currently ' + str(current_temp) + ' degrees fahrenheit')
print('Stick a fork in me, I am done!')
elif current_temp > pork_cooked :
print('Oh no! I am overcooked by ' + str(current_temp - pork_cooked) + ' degrees')
break
current_temp = current_temp + 12
else: print('I do not know how to cook this meat')
return current_temp
cooking('steak')
This was by far the best and most fun topic. Error Handling
Why?
Because I get to intentionally break things to see the innerworkings of Python
💬 I like to say: failure is the best way to learn something. Specifically with coding, you can learn so much from researching a particular error message to see why things work the way they work.
I stepped away from my chicken cooking program, I wanted to cook dessert instead 🥧
This function calculates how many pies to cook given the parameter, which is the number of people to feed.
I had to put a cheezy disclaimer here that it assumes a pie feeds 3.14 people... get it 😜
## Error Handling
def pie_calculation(people):
''' This function will calculate how many pies to cook given the parameter,
which is the number of people to feed
NOTE: this function assumes that a pie feeds 3.14 people, hehe '''
try:
if(people < 0):
raise ValueError('Number of people cannot be negative')
return round(people / 3.14)
except TypeError:
print('Number of people must be an int or float')
pie_calculation(2)
pie_calculation('two')
pie_calculation(-5)
pie_calculation(20)
Below is a screen of the output from the above script. This is Spyder, an IDE for Python. I like this tool because it feels familiar. I started my programming career learning R and it feels a lot like RStudio.
Other tools I have used for Python are Jupiter Notebooks and PyCharm.
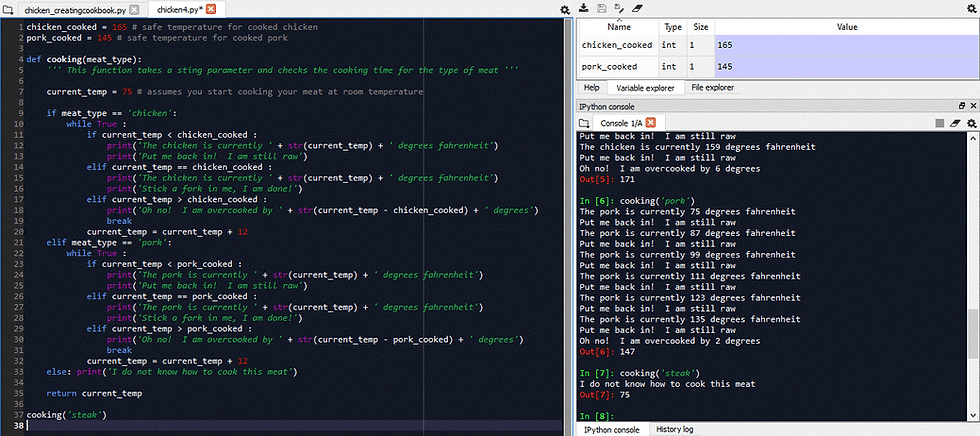
Comments